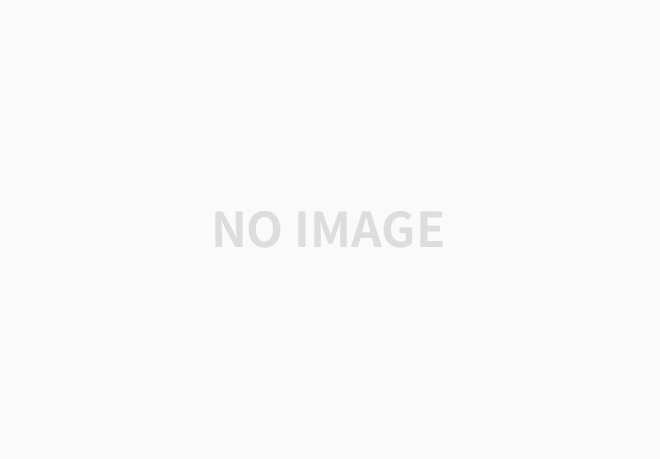
안녕하세요. 후르륵짭짭입니다.
작년에 나온 기술인데, 이제 경험 해보네요 ㅋㅋㅋ
실제로 프로젝트에 적용해보지 않아서 얼마나 강력한지 모르지만
새로운 Concurrency 기술이 궁금하여 경험해봤습니다.
** DispatchQueue를 대신하는 새로운 비동기 기술 **
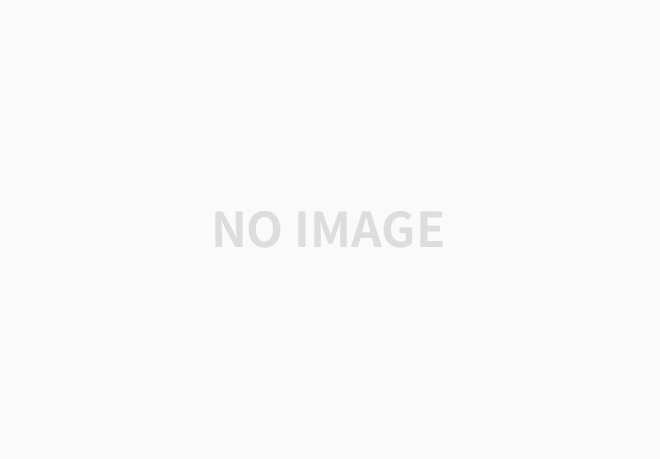
해당 링크에 자세하게 Swift Concurrency에 대해 설명을 하고 있습니다.
https://developer.apple.com/videos/play/wwdc2021/10132
Meet async/await in Swift - WWDC21 - Videos - Apple Developer
Swift now supports asynchronous functions — a pattern commonly known as async/await. Discover how the new syntax can make your code...
developer.apple.com
** Command Line Tool에서 테스트 해보기 **
일단 Async - Await를 PlayGround에서 완벽하게 검증하기 어려웠다.
아직 PlayGround에서는 이슈가 존재하는 것 같다.
그래서 Command Line Tool을 이용하려 했는데, Command Line Tool에서는 Sync Main Thread를 일회성으로 사용해서
@main을 지정해줘야 Async로 돌아가는 Thread를 사용할 수 있다.
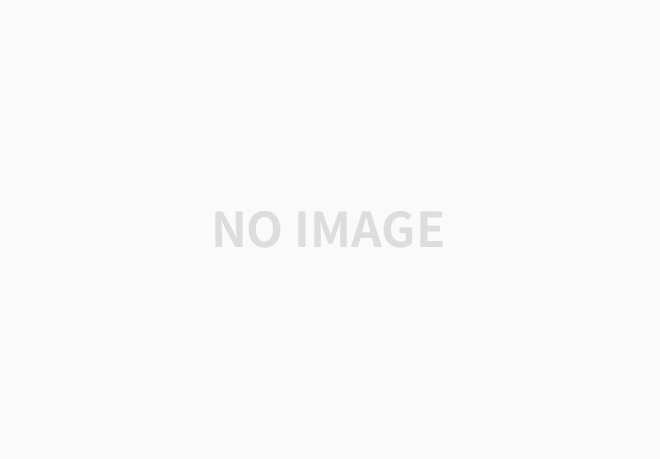
** 순차적으로 동작하는 Async Await 지정 **
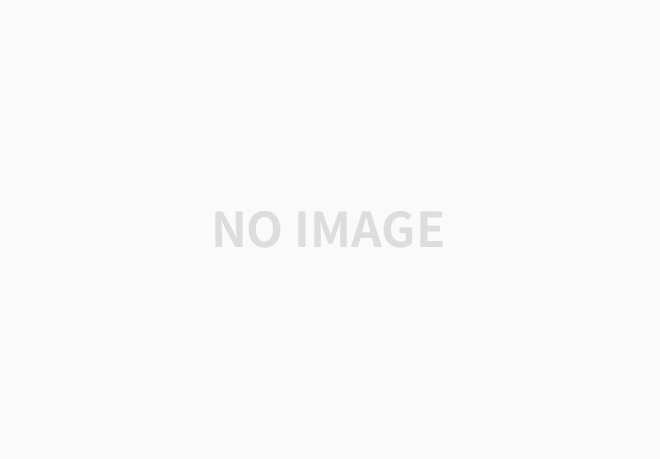
가장 기본적인 Code를 보면
func fetchFirstString() async -> String {
Thread.sleep(forTimeInterval: 3)
print("fetchFirstString Called")
return "Hello ChapChap"
}
위와 같이 async라는 표시를 해준다.
그리고 Return 값을 설정해준다. (Throws랑 똑같다....)
이것은 해당 함수는 비동기 작업을 하기 때문에 결과값이 언제 나올 지 모르고 결과가 나온다면 String으로 반환한다는 것을 의미한다.
func processWithSequence(){
Task{
let userId = await fetchUserID(userID: 20)
let firstString = await fetchFirstString()
greeting = firstString + " : " + userId
/*
Hello, playground
Hello, playground
fetchUserID Called
fetchFirstString Called
Hello ChapChap : 20
*/
printGreeting()
}
printGreeting()
}
위 코드를 보면 async라는 표시를 한 함수를 사용할 때
이 함수는 비동기작업이기 때문에 기다리도록 한다라는 표시로 await를 함수 앞에 작성해준다.
그러면 순차적으로 fetchUserId -> fetchFirstString 함수를 수행하고 printGreeting을 수행하게 된다.
참고로 await를 사용하기 위해서는 비동기 작업 환경이 구축된 곳에서 사용 가능하다.
따라서 함수에 async가 적혀 있거나, Task를 통해서 해당 작업이 비동기 라는 것을 명시해줘야 사용가능하다.
** 병렬적으로 작업하는 Async **
DispatchGroup이라고 불리는 기능도 Swift Concurrency에서 지원한다.
func processWithParallel() {
Task{
async let userId = fetchUserID(userID: 20)
async let firstString = fetchFirstString()
/*
Hello, playground
fetchFirstString Called
fetchUserID Called
Hello ChapChap 20
*/
await print(firstString , userId)
}
}
방법은 동일하나 async let 으로 바꿔주면 된다.
** Error를 반환하는 Async Await **
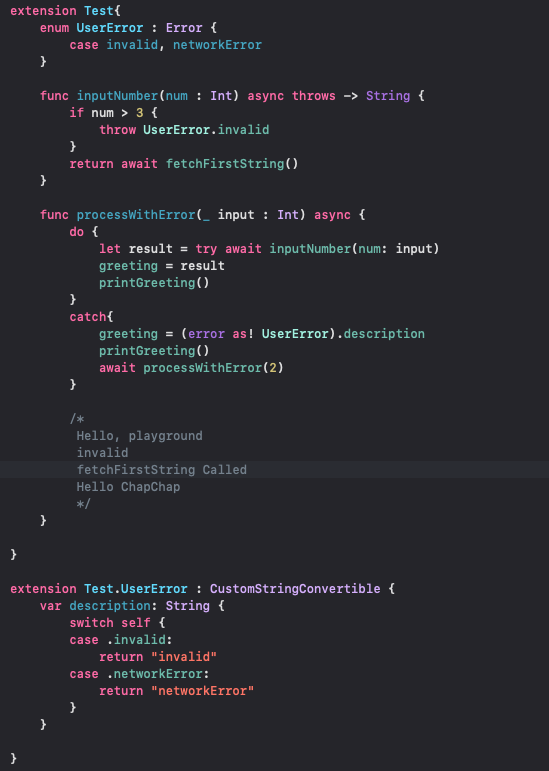
일반적인 에러 처리 방법과 동일하다.
그냥 async에 throws를 적어주면 끝이다.
** Complete Handler와 Async Await를 함께 **
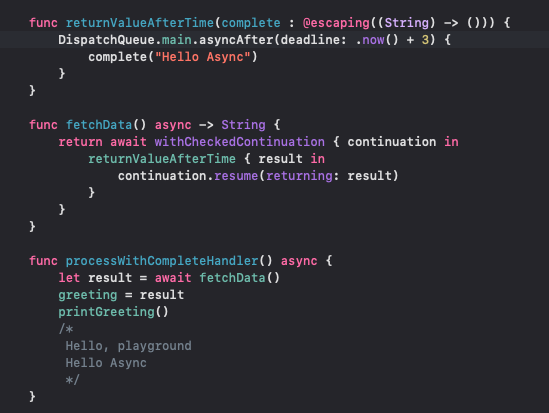
위와 같이 withCheckedContinuation을 통해서 기존의 CompleteHandler의 비동기 처리를 Async로 바꿀 수 있다.
하지만 withCheckedContinuation를 사용할 때 반드시
resume을 무조건 한번 사용해야한다.
그 이상을 사용하면 Crash가 발생하고 하나도 사용하지 않는다면 결과값이 반환되지 않고 무한정 Await하는 현상이 발생한다.
** 참고 사이트 **
Async/Await 사용 한국
Swift Concurrency에 대해서
2022-LINE-engineering-site
engineering.linecorp.com
https://ios-development.tistory.com/958
[iOS - swift] Async, Await 사용 방법
Async, Await 이란? 기존에 비동기 처리 방식은 DispatchQueue나 completionHandler를 사용하여 처리했지만, 더욱 편하게 비동기 처리할 수 있는 문법 // DispatchQueue 사용한 비동기 처리 DispatchQueue.global...
ios-development.tistory.com
[CS193p] GCD를 async/await로 변경하기
CS193p 강의의 lec 9, 10에서 GCD를 사용한 것을 new async await로 변경해보겠습니다. 먼저 강의에서 언급한 Swift's new built-in async API는 async/await를 지칭하는 것 같습니다. 2021 WWDC - Meet async/aw..
skagh.tistory.com
https://blog.slarea.com/swift/5-5/async-await/
[Swift 5.5] async await
Javascript, kotlin, google-promises 등 그 동안 다른 언어에서 제공되던 기능이 Swift 5.5 에 강력하게 들어왔다. 비동기 프로그래밍은 그동안 delegate 패턴이나, closur 를 통해 해왔다면 앞으로는 async, await
blog.slarea.com
Async/Await 사용 영어
https://www.hackingwithswift.com/swift/5.5/async-await
Async await – available from Swift 5.5
Link copied to your pasteboard.
www.hackingwithswift.com
https://www.hackingwithswift.com/articles/233/whats-new-in-swift-5-5
What's new in Swift 5.5?
Async/await, actors, throwing properties, and more!
www.hackingwithswift.com
https://wwdcbysundell.com/2021/wrapping-completion-handlers-into-async-apis/
Wrapping completion handlers into async APIs | WWDC by Sundell & Friends
If you've been following the WWDC sessions about Swift’s new async/await feature, then you probably already know that most of the asynchronous APIs within Apple’s SDK that used to return their results through completion handlers now offer brand new asy
wwdcbysundell.com
Main Actor와 Task
https://www.andyibanez.com/posts/mainactor-and-global-actors-in-swift/
@MainActor and Global Actors in Swift
Learn what @MainActor is and how you can use Global Actors in Swift.
www.andyibanez.com
How can I convert to Swift async/await from GCD (DispatchQueue)?
I am following Stanfords' CS193p Developing Apps for iOS online course. It is using the Grand Central Dispatch (GCD) API for a demo of multithreading. But they noted, that "GCD has been mostly
stackoverflow.com
Async가 command Line Tool에서 작동 안 할 때
'async' call in a function that does not support concurrency swift ios Xcode async/await
I'm trying to use async/await with Swift 5.5. I have my async function, but whenever I try to call it, I get this error: 'async' call in a function that does not support concurrency Here's the c...
stackoverflow.com
Async Await를 일반 함수에서 사용할 때 발생하는 에러
How to fix the error “async call in a function that does not support concurrency” - a free Swift Concurrency by Example tuto
Was this page useful? Let us know! 1 2 3 4 5
www.hackingwithswift.com
Continuouse와 unsafe의 차이
https://www.donnywals.com/the-difference-between-checked-and-unsafe-continuation-in-swift/
The difference between checked and unsafe continuations in Swift – Donny Wals
When you’re writing a conversion layer to transform your callback based code into code that supports async/await in Swift, you’ll typically find yourself using continuations. A continuation is a…
www.donnywals.com
'Xcode > Swift - PlayGround' 카테고리의 다른 글
PlayGround) Swift Combine 적응기 #1 (Custom Publisher) (0) | 2022.11.05 |
---|---|
PlayGround) Objective-C (상속, 카테고리, 프로토콜, 구조체) 정리 (1) | 2022.09.26 |
PlayGround) RxTest에서 Timer들어간 Observable 테스트 (0) | 2022.04.24 |
PlayGround) Subscript란? (0) | 2022.03.15 |
PlayGround) Serial에서 Async는 머지? (0) | 2022.02.28 |
댓글